Display Net amount to the order totals in Magento 2
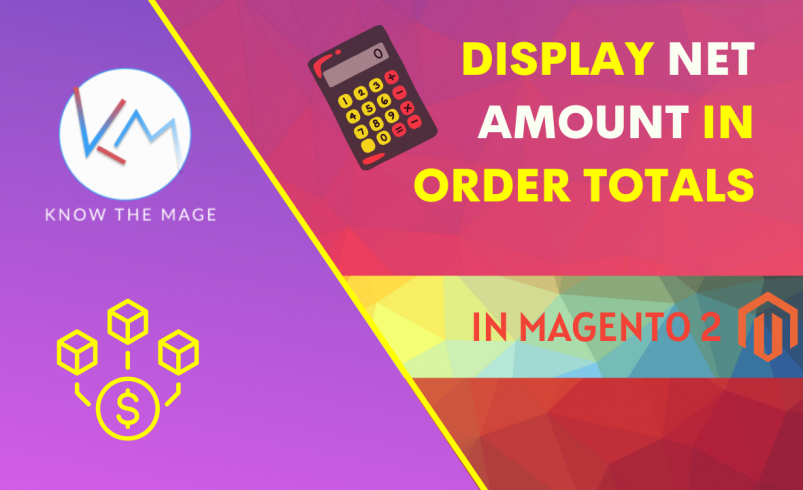
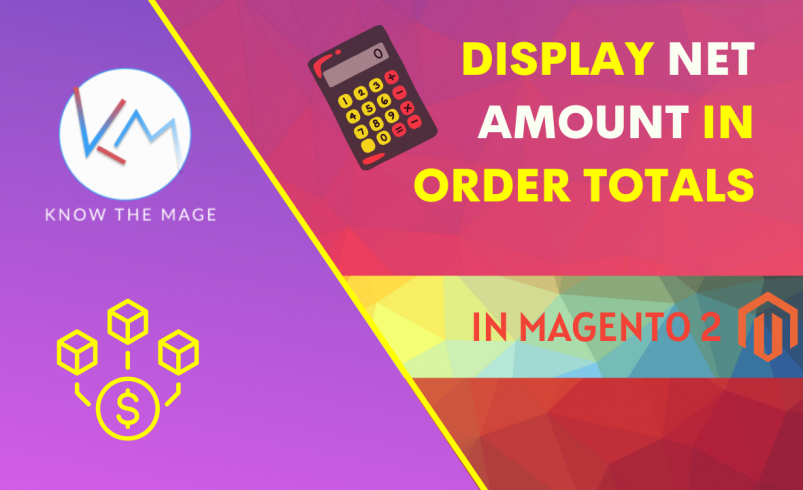
Today we will talk about adding a custom field between the order totals. This field calculates all totals except the tax amount. We call this field as Net Amount. The net amount is sum of subtotals , shipping amount and deduces from discount amount.
Begin with creating checkout_cart_index.xml layout and pushing the netamount block totals. Know/Module/view/frontend/layout/checkout_cart_index.xml
<?xml version="1.0"?> <page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd"> <body> <referenceBlock name="checkout.cart.totals"> <arguments> <argument name="jsLayout" xsi:type="array"> <item name="components" xsi:type="array"> <item name="block-totals" xsi:type="array"> <item name="children" xsi:type="array"> <item name="netamount" xsi:type="array"> <item name="component" xsi:type="string">Know_Module/js/view/checkout/cart/totals/net-amount</item> <item name="sortOrder" xsi:type="string">40</item> <item name="config" xsi:type="array"> <item name="template" xsi:type="string">Know_Module/checkout/cart/totals/net-amount</item> <item name="title" xsi:type="string" translate="true">Net Amount</item> </item> </item> </item> </item> </item> </argument> </arguments> </referenceBlock> </body> </page>
Now we will create a netamount js component. Know/Module/view/frontend/web/js/view/checkout/cart/totals/net-amount.js
/*global alert*/ define([ 'Know_Module/js/view/checkout/summary/net-amount' ], function (Component) { 'use strict'; return Component.extend({ /** * @override * use to define amount is display setting */ isDisplayed: function () { return true; } }); });
Create net-amount template: Know/Module/view/frontend/web/template/checkout/cart/totals/net-amount.html
<tr class="totals netamount excl"> <th class="mark" colspan="1" scope="row" data-bind="text: title"></th> <td class="amount"> <span class="price" data-bind="text: getValue()"></span> </td> </tr>
Create Know_Module/js/view/checkout/summary/net-amount summary component: Know/Module/view/frontend/web/js/view/checkout/summary/net-amount.html
/*global alert*/ define([ 'Magento_Checkout/js/view/summary/abstract-total', 'Magento_Checkout/js/model/quote', 'Magento_Catalog/js/price-utils', 'Magento_Checkout/js/model/totals' ], function ( Component, quote, priceUtils, totals ) { "use strict"; return Component.extend({ defaults: { isFullTaxSummaryDisplayed: window.checkoutConfig.isFullTaxSummaryDisplayed || false, template: 'Know_Module/checkout/summary/net-amount' }, totals: quote.getTotals(), isTaxDisplayedInGrandTotal: window.checkoutConfig.includeTaxInGrandTotal || false, isDisplayed: function() { return this.isFullMode(); }, getValue: function() { var price = 0; if (this.totals()) { if (totals.getSegment('subtotal_with_discount')) { price = parseFloat(totals.getSegment('subtotal_with_discount').value); } else { price = parseFloat(this.totals().subtotal_with_discount); } // Include store cash amount if (totals.getSegment('store_payments')) { price += parseFloat(totals.getSegment('store_payments').value); } else { price += parseFloat(this.totals().store_payments !== undefined ? this.totals().store_payments : 0); } if (totals.getSegment('shipping')) { price += parseFloat(totals.getSegment('shipping').value); } else { price += parseFloat(this.totals().shipping); } } return this.getFormattedPrice(price); }, getBaseValue: function() { var price = totals.getBaseValue(); if (this.totals()) { price = this.totals().base_subtotal_with_discount; } return priceUtils.formatPrice(price, quote.getBasePriceFormat()); } }); });
Create summary net-amount template: Know/Module/view/frontend/web/template/checkout/summary/net-amount.html
<tr class="totals netamount excl"> <th class="mark" scope="row"> <span class="label" data-bind="text: title"></span> <span class="value" data-bind="text: getValue()"></span> </th> <td class="amount"> <span class="price" data-bind="text: getValue(), attr: {'data-th': title}"></span> </td> </tr>
Run setup upgrade command to install module:
php bin/magento setup:upgrade
Add items to cart and navigate to cart page. To observe net amount calculations try with applying coupons and shipping fee.
The cart totals:
Next step is displaying the Net amount at checkout totals section. To do so we need to specify the netamount components inside checkout_index_index.xml layout file under sidebar node.
Create checkout_index_index.xml layout: Know/Module/view/frontend/layout/checkout_index_index.xml
<?xml version="1.0"?> <page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd"> <body> <referenceBlock name="checkout.root"> <arguments> <argument name="jsLayout" xsi:type="array"> <item name="components" xsi:type="array"> <item name="checkout" xsi:type="array"> <item name="children" xsi:type="array"> <item name="sidebar" xsi:type="array"> <item name="children" xsi:type="array"> <item name="summary" xsi:type="array"> <item name="children" xsi:type="array"> <item name="totals" xsi:type="array"> <item name="children" xsi:type="array"> <item name="netamount" xsi:type="array"> <item name="component" xsi:type="string">Know_Module/js/view/checkout/cart/totals/net-amount</item> <item name="sortOrder" xsi:type="string">40</item> <item name="config" xsi:type="array"> <item name="template" xsi:type="string">Know_Module/checkout/cart/totals/net-amount</item> <item name="title" xsi:type="string" translate="true">Net Amount</item> </item> </item> </item> </item> </item> </item> </item> </item> </item> </item> </item> </argument> </arguments> </referenceBlock> </body> </page>
We already have created the component and relating template file. Now flush the cache:
php bin/magento c:c
Navigate to the checkout page and to the payment step. The order totals now include the Net amount. Make sure the calculations are correct. The output will be similar to the image blow:
Next we will add the Net Amount to the customer order detail page.
Go a head and create sales_order_view.xml layout file under frontend directory: Know/Module/view/frontend/layout/sales_order_view.xml
Add following snippet to the layout:
<?xml version="1.0"?> <page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd"> <body> <referenceContainer name="order_totals"> <block class="Know\Module\Block\Sales\Totals\NetAmount" name="netamount" /> </referenceContainer> </body> </page>
Create the NetAmount block class: Know/Module/Block/Sales/Totals/NetAmount
<?php namespace Know\Module\Block\Sales\Totals; use Magento\Framework\View\Element\Template; use Magento\Framework\View\Element\Template\Context; use Magento\Sales\Model\Order; class NetAmount extends Template { const CODE = 'netamount'; /** * Tax configuration model * * @var \Magento\Tax\Model\Config */ protected $_config; /** * @var Order */ protected $_order; /** * @var \Magento\Framework\DataObject */ protected $_source; /** * @param Context $context * @param \Magento\Tax\Model\Config $taxConfig * @param array $data */ public function __construct( Context $context, \Magento\Tax\Model\Config $taxConfig, array $data = [] ) { $this->_config = $taxConfig; parent::__construct($context, $data); } /** * Check if we nedd display full tax total info * * @return bool */ public function displayFullSummary() { return true; } /** * Get data (totals) source model * * @return \Magento\Framework\DataObject */ public function getSource() { return $this->_source; } /** * @return \Magento\Store\Model\Store */ public function getStore() { return $this->_order->getStore(); } /** * @return Order */ public function getOrder() { return $this->_order; } /** * @return array */ public function getLabelProperties() { return $this->getParentBlock()->getLabelProperties(); } /** * @return array */ public function getValueProperties() { return $this->getParentBlock()->getValueProperties(); } /** * Initialize all order totals relates with tax * * @return \Magento\Tax\Block\Sales\Order\Tax|$this */ public function initTotals() { $parent = $this->getParentBlock(); $this->_order = $parent->getOrder(); $this->_source = $parent->getSource(); $netamount = new \Magento\Framework\DataObject( [ 'code' => self::CODE, 'strong' => false, 'value' => $this->_source->getData('subtotal') + $this->_source->getData('discount_amount') + $this->_source->getData('shipping_amount'), 'base_value' => $this->_source->getData('base_subtotal') + $this->_source->getData('base_discount_amount') + $this->_source->getData('base_shipping_amount'), 'label' => __('Net Amount (Excluding Tax)') ] ); $parent->addTotal($netamount, self::CODE); $parent->addTotalBefore($netamount, 'grand_total'); return $this; } }
The output for order totals:
Add Net Amount to the invoice totals. Create the sales_order_invoice.xml layout: Know/Module/view/frontend/layout/sales_order_invoice.xml
<?xml version="1.0"?> <page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd"> <body> <referenceContainer name="invoice_totals"> <block class="Know\Module\Block\Sales\Totals\NetAmount" name="netamount" /> </referenceContainer> </body> </page>
Next add the Net Amount to the creditmemo totals. Create layout: Know/Module/view/frontend/layout/sales_order_creditmemo.xml
<?xml version="1.0"?> <page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd"> <body> <referenceContainer name="creditmemo_totals"> <block class="Know\Module\Block\Sales\Totals\NetAmount" name="netamount" /> </referenceContainer> </body> </page>
Display the Net Amount to the print order totals. Create sales_order_print.xml layout and add NetAmount block to the order_totals container: Know/Module/view/frontend/layout/sales_order_print.xml
<?xml version="1.0"?> <page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd"> <body> <referenceContainer name="order_totals"> <block class="Know\Module\Block\Sales\Totals\NetAmount" name="netamount" before="order_totals" /> </referenceContainer> </body> </page>
Include the Net Amount in email order totals. Create sales_email_order_items.xml layout and add the NetAmount block to it: Know/Module/view/frontend/layout/sales_email_order_items.xml
<?xml version="1.0"?> <page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd"> <body> <referenceContainer name="order_totals"> <block class="Know\Module\Block\Sales\Totals\NetAmount" name="netamount" /> </referenceContainer> </body> </page>